Steps to select an element in Angular component template
- Add a template reference variable to the component HTML element.
- Import
@ViewChild
decorator from@angular/core
in component ts file. - Use
ViewChild
decorator to access template reference variable inside the component. - And finally select the element in
ngAfterViewInit
method by accessing element’snativeElement
property.
Now we will go through an example to understand it further.
We will create a component called select-element
in our angular project.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-select-element',
templateUrl: './select-element.component.html',
styleUrls: ['./select-element.component.scss']
})
export class SelectElementComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
In component html file I have added a input
element with template reference variable #inputElement
<input #inputElement value="Angular Wiki">
In component file we can access #inputElement
by using @ViewChild
decorator.
import { Component, OnInit, ViewChild, ElementRef }
from '@angular/core';
@Component({
selector: 'app-select-element',
templateUrl: './select-element.component.html',
styleUrls: ['./select-element.component.scss']
})
export class SelectElementComponent implements OnInit {
@ViewChild('inputElement') inputElement:ElementRef;
constructor() { }
ngOnInit() {
}
ngAfterViewInit() {
console.log(this.inputElement.nativeElement.value);
}
}
In ngAfterViewInit
method I am logging the value of inputElement i.e., “Angular Wiki”
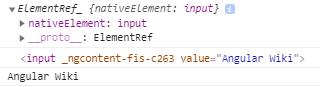